lexikos wrote:giving up certain features
Yes, I'm starting to do that. For example, one program has a feature to create a tray icon report, either for all icons in the tray or just for that program's icons (there can be many). The report contains each icon's position number in the tray, its owning process, tooltip, and which tray it is in (Shell_TrayWnd or NotifyIconOverflowWindow). It's a useful report, but at this point, I'm willing to give it up on W11.
lexikos wrote:or supporting only older builds
Agreed. For example, I may keep the tray icon report on earlier W11 builds and all W10 (and prior) releases.
lexikos wrote:These are tray icons created by your scripts?
Each instance of the program has its own icon. For example, one program has various color stars in the tray, such as Blue, Green, Red, Yellow (and lots more):
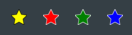
- color star tray icons.png (2.16 KiB) Viewed 6194 times
Another program allows the user to toggle Caps Lock, Num Lock, and Scroll Lock via tray icons. Each key's icon is red when it's off and green when it's on:

- caps num scroll lock tray icons.png (1.42 KiB) Viewed 6194 times
Both of those programs have a feature that allows the user to move the icons in the tray. For example, the Caps Lock, Num Lock, and Scroll Lock icons have this context submenu hanging off a Tools menu:

- move tray icons.png (2.08 KiB) Viewed 6194 times
The process name is the same for all instances of the same program. The various programs are compiled with Ahk2Exe and they all have an installer (a Setup.exe file) created with NSIS.
lexikos wrote:Why automate the tray icons when you can automate the script itself?
The programs have plenty of automation, but I chose to have the UI for these programs center around the context menus of the system tray icons, although many of the context menu choices lead to "normal" windows, many of which contain GUIs, ListViews, etc.
lexikos wrote:You can show a script's tray menu by sending it the same notification message that the tray would send: AHK_NOTIFYICON (1028) with lParam = WM_RBUTTONUP (0x205).
That seems to be what the
TrayIcon_Button function does, which is not working on the recent W11 builds. It would be great if I can assign a hotkey to that for each instance, such as
Alt+Ctrl+B to open the context menu of the Blue star.
lexikos wrote:Maybe that's not an issue if the tooltips are unique.
Correct...not an issue...each running instance has a unique tooltip.
lexikos wrote:The user can still re-order them with drag-drop
Yes. That had stopped working in W11 Build 22581, but Microsoft fixed it. Interesting thread with Microsoft on that issue (8 months ago):
Microsoft Response: We'll be continuing to monitor this feedback, but with the updates we made for the new tablet-optimized taskbar in Build 22563, we're no longer supporting dragging icons in the system tray or between the system tray and the show hidden icons flyout. Instead, you should use the Settings > Personalization > Taskbar > System tray section to manage these icons.
UPDATE: We have decided to disable the changes to the system tray introduced in Build 22581 for now. The system tray and the Show hidden icons flyout will now function the same way it did with the original release of Windows 11, including the ability to rearrange icons in the flyout. We hope to bring these changes back in the future after further refinement of the experience by addressing some of this feedback. This is now available with Build 22616, now available in the Dev and Beta Channels:
https://aka.ms/wip22616
lexikos wrote:as far as I can tell, the order persists across reboots
I don't know...haven't tested that.
lexikos wrote:but maybe for separate compiled scripts
As noted above, each of my programs is a separately compiled script, each with its own (Setup.exe) installer, so that may work.
Thanks for all your thoughts on this...much appreciated! Regards, Joe