I want to create a gui which shows this picture:
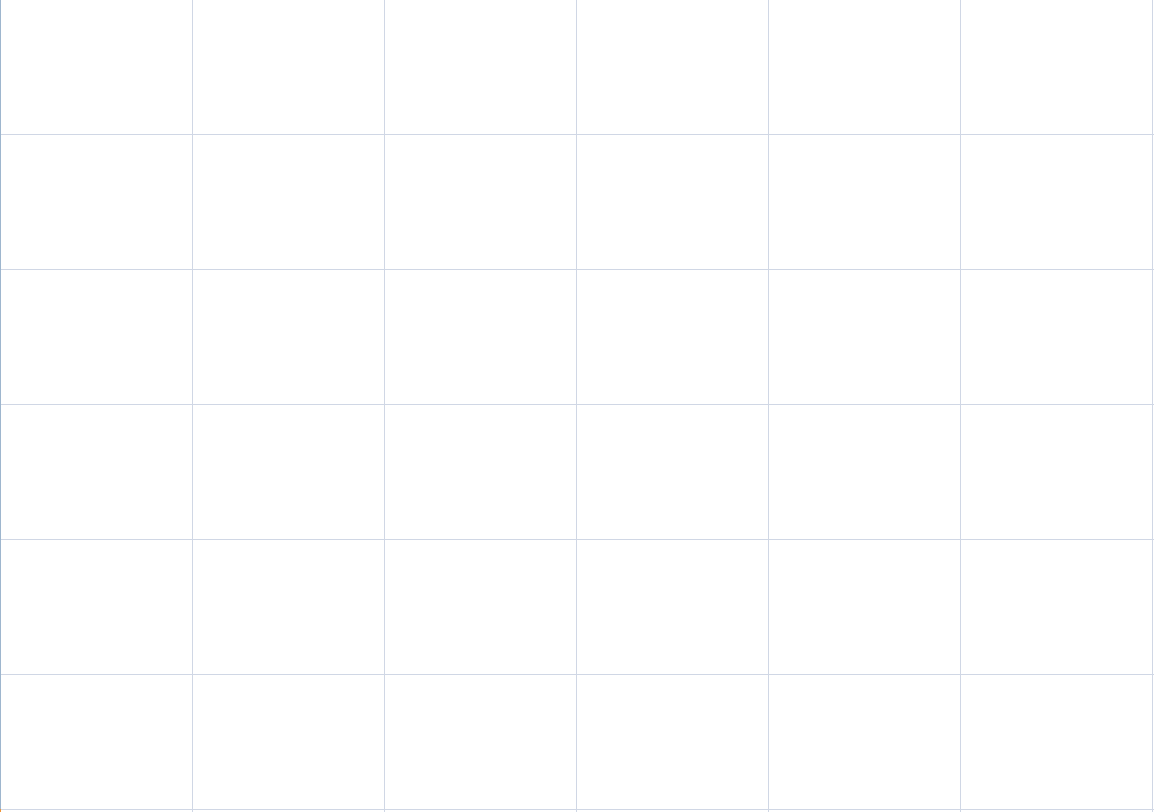
The code will probably be something like:
Code: Select all
Gui, -Caption
Gui, +AlwaysOnTop
Gui, Show, x0 y0 w1920 h1080
Gui, Add, Picture, x0 y0 w1920 h1080, picture.png
return
(But let's ignore the coloring function at the moment...)
I believe it's correct/helpful that all squares should be defined as array elements.
A1, B1, C1, D1 etc.
In the AHK tutorial I read that associative arrays contain both elements and values, which are linked to the elements.
Like:
Code: Select all
array := {ten: 10, twenty: 20, thirty: 30}
It should be something like:
squareArray := {A1: x = 0..100 y = 0..100, B1: x = 101..200 y = 101..200}
Whenever I click with the left mouse button inside of a square, AHK would check the x/y-coordinates.
if x is in the range of 0 till 100 and y is in the range of 0 till 100, it would mean the square is A1.
I know that using arrays isn't necessary for the functionality, because I already got it work without arrays,
but using arrays might give me more possibilities, especially when I want to colorize a square.
Code: Select all
lbutton:: ; Left-click
MouseGetPos, xx, yy ; Check current mouse position
if (xx < 128) & (yy < 128)
{
MsgBox, A1
}
else if (xx < 256) & (yy < 128)
{
MsgBox, B1
}
else if (xx < 384) & (yy < 128)
{
MsgBox, C1
}
etc.
1. Is it meaningful to work with (associative) arrays here? (As mentioned, I would like to colorize the squares later...)
2. How would I specify that A1 is from x = 0 till 100 and y = 0 till 100?
Thanks for any help!